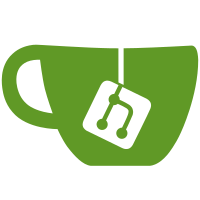
This is an attempt to make boost::atomic<> interface closer to the standard. It makes a difference in C++17 as it mandates copy elision, which makes this code possible: boost::atomic<int> a = 10; It also makes is_convertible<T, boost::atomic<T>> return true, which has implications on the standard library components, such as std::pair. This removes the workaround for gcc 4.7, which complains that operator=(value_arg_type) is considered ambiguous with operator=(atomic const&) in assignment expressions, even though conversion to atomic<> is less preferred than conversion to value_arg_type. We try to work around the problem from the operator= side. Added a new compile test to check that the initializing constructor is implicit.
35 lines
946 B
C++
35 lines
946 B
C++
// Copyright (c) 2018 Andrey Semashev
|
|
//
|
|
// Distributed under the Boost Software License, Version 1.0.
|
|
// See accompanying file LICENSE_1_0.txt or copy at
|
|
// http://www.boost.org/LICENSE_1_0.txt)
|
|
|
|
// The test verifies that atomic<T> has an implicit conversion constructor from T.
|
|
// This can only be tested in C++17 because it has mandated copy elision. Previous C++ versions
|
|
// also require atomic<> to have a copy or move constructor, which it does not.
|
|
#if __cplusplus >= 201703L
|
|
|
|
#include <boost/atomic.hpp>
|
|
#include <boost/static_assert.hpp>
|
|
#include <boost/config.hpp>
|
|
#include <type_traits>
|
|
|
|
int main(int, char *[])
|
|
{
|
|
static_assert(std::is_convertible< int, boost::atomic< int > >::value, "boost::atomic<T> does not have an implicit constructor from T");
|
|
|
|
boost::atomic< short > a = 10;
|
|
(void)a;
|
|
|
|
return 0;
|
|
}
|
|
|
|
#else // __cplusplus >= 201703L
|
|
|
|
int main(int, char *[])
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
#endif // __cplusplus >= 201703L
|