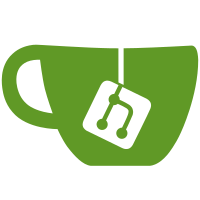
Split header tests accordingly i.e. test core headers as part of
core tests, numeric extension headers as part of numeric tests, etc.
It extends the convention of sub-directories already established in
`include/boost/gil` directory. It is sensible to follow it in other
areas of the source tree (i.e. `test/`, `doc/` and `benchmark/`).
Another important reason to move the tests is to enable removal of
the top-level `Jamfile` with all its definitions of test-specific
requirements.
The top-level `Jamfile` is not advised, especially if it specifies
build requirements like C++ language version.
Those affect non-tests builds e.g. documentation, causing failures
during generation of HTML documentation (leads to missing docs).
(cherry picked from develop branch commit 4ed7701b47
)
82 lines
2.8 KiB
C++
82 lines
2.8 KiB
C++
//
|
|
// Copyright 2018 Mateusz Loskot <mateusz at loskot dot net>
|
|
//
|
|
// Distributed under the Boost Software License, Version 1.0
|
|
// See accompanying file LICENSE_1_0.txt or copy at
|
|
// http://www.boost.org/LICENSE_1_0.txt
|
|
//
|
|
#include <limits>
|
|
|
|
#define BOOST_TEST_MODULE test_channel_test_fixture
|
|
#include "unit_test.hpp"
|
|
#include "test_fixture.hpp"
|
|
|
|
namespace fixture = boost::gil::test::fixture;
|
|
|
|
BOOST_AUTO_TEST_CASE_TEMPLATE(channel_minmax_value_integral, Channel, fixture::channel_integer_types)
|
|
{
|
|
fixture::channel_minmax_value<Channel> fix;
|
|
fixture::channel_minmax_value<Channel> exp;
|
|
BOOST_TEST(fix.min_v_ == exp.min_v_);
|
|
BOOST_TEST(fix.max_v_ == exp.max_v_);
|
|
BOOST_TEST(fix.min_v_ == std::numeric_limits<Channel>::min());
|
|
BOOST_TEST(fix.max_v_ == std::numeric_limits<Channel>::max());
|
|
}
|
|
|
|
BOOST_AUTO_TEST_CASE_TEMPLATE(channel_minmax_value_float, Channel, fixture::channel_float_types)
|
|
{
|
|
fixture::channel_minmax_value<Channel> fix;
|
|
fixture::channel_minmax_value<Channel> exp;
|
|
BOOST_TEST(fix.min_v_ == exp.min_v_);
|
|
BOOST_TEST(fix.max_v_ == exp.max_v_);
|
|
}
|
|
|
|
BOOST_AUTO_TEST_CASE_TEMPLATE(channel_value, Channel, fixture::channel_byte_types)
|
|
{
|
|
fixture::channel_value<Channel> fix;
|
|
fixture::channel_minmax_value<Channel> exp;
|
|
BOOST_TEST(fix.min_v_ == exp.min_v_);
|
|
BOOST_TEST(fix.max_v_ == exp.max_v_);
|
|
}
|
|
|
|
BOOST_AUTO_TEST_CASE_TEMPLATE(channel_reference, Channel, fixture::channel_byte_types)
|
|
{
|
|
fixture::channel_reference<Channel&> fix;
|
|
fixture::channel_minmax_value<Channel> exp;
|
|
BOOST_TEST(fix.min_v_ == exp.min_v_);
|
|
BOOST_TEST(fix.max_v_ == exp.max_v_);
|
|
}
|
|
|
|
BOOST_AUTO_TEST_CASE_TEMPLATE(
|
|
channel_reference_const, Channel, fixture::channel_byte_types)
|
|
{
|
|
fixture::channel_reference<Channel const&> fix;
|
|
fixture::channel_minmax_value<Channel> exp;
|
|
BOOST_TEST(fix.min_v_ == exp.min_v_);
|
|
BOOST_TEST(fix.max_v_ == exp.max_v_);
|
|
}
|
|
|
|
BOOST_AUTO_TEST_CASE_TEMPLATE(
|
|
packed_channels565, BitField, fixture::channel_bitfield_types)
|
|
{
|
|
static_assert(std::is_integral<BitField>::value, "bitfield is not integral type");
|
|
|
|
// Regardless of BitField buffer bit-size, the fixture is initialized
|
|
// with max value that fits into 5+6+5 bit integer
|
|
fixture::packed_channels565<BitField> fix;
|
|
fixture::channel_minmax_value<std::uint16_t> exp;
|
|
BOOST_TEST(fix.data_ == exp.max_v_);
|
|
}
|
|
|
|
BOOST_AUTO_TEST_CASE_TEMPLATE(
|
|
packed_dynamic_channels565, BitField, fixture::channel_bitfield_types)
|
|
{
|
|
static_assert(std::is_integral<BitField>::value, "bitfield is not integral type");
|
|
|
|
// Regardless of BitField buffer bit-size, the fixture is initialized
|
|
// with max value that fits into 5+6+5 bit integer
|
|
fixture::packed_dynamic_channels565<BitField> fix;
|
|
fixture::channel_minmax_value<std::uint16_t> exp;
|
|
BOOST_TEST(fix.data_ == exp.max_v_);
|
|
}
|