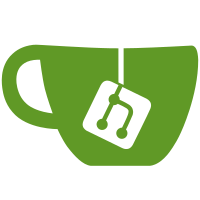
* Implement Sobel and Scharr operators This commit adds Sobel and Scharr operators with support for 0th and 1st degrees with other degrees planned for later * Migrate and fix Harris example Generate Harris entries now uses signed image view. The Harris corner detector example now uses the Scharr filter generator and convolve_2d to reduce amount of code needed. * Fix and migrate Hessian example The Hessian example now uses signed image views and uses newly added kernel generators to compute gradients * Fix Harris and Hessian tests The tests broke due to migration to signed views in algorithms, but tests were not adjusted * Fix Jamfile for example/sobel_scharr.cpp * Cosmetic changes * Commented out fail tests * Fixed pixel16 used in image16s In Harris and Hessian tests, unsigned pixel values was used to construct signed image, which was causing appveyor to error out. * Reenable failing targets * Unify kernel generator interface This commit makes all kernel generator functions to return kernel_2d and adapts dependant threshold function to use the new interface * Migrate Hessian and Harris tests Migrate Hessian and Harris tests to new interface for kernel generators * Migrate Harris and Hessian examples Harris and Hessian examples now use new interface for kernel generation * Migrate simple_kernels tests simple_kernels are now using kernel_2d interface * Add missing return Normalized mean generation had missing return at the end of the function * Adapt code to namespace move This commit reacts to kernel_2d, convolve_2d being moved to namespace detail
73 lines
2.2 KiB
C++
73 lines
2.2 KiB
C++
//
|
|
// Copyright 2019 Olzhas Zhumabek <anonymous.from.applecity@gmail.com>
|
|
//
|
|
// Use, modification and distribution are subject to the Boost Software License,
|
|
// Version 1.0. (See accompanying file LICENSE_1_0.txt or copy at
|
|
// http://www.boost.org/LICENSE_1_0.txt)
|
|
//
|
|
#include <boost/core/lightweight_test.hpp>
|
|
#include <boost/gil/image.hpp>
|
|
#include <boost/gil/image_view.hpp>
|
|
#include <boost/gil/image_processing/numeric.hpp>
|
|
#include <boost/gil/image_processing/harris.hpp>
|
|
|
|
namespace gil = boost::gil;
|
|
|
|
bool are_equal(gil::gray32f_view_t expected, gil::gray32f_view_t actual) {
|
|
if (expected.dimensions() != actual.dimensions())
|
|
return false;
|
|
|
|
for (long int y = 0; y < expected.height(); ++y)
|
|
{
|
|
for (long int x = 0; x < expected.width(); ++x)
|
|
{
|
|
if (expected(x, y) != actual(x, y))
|
|
{
|
|
return false;
|
|
}
|
|
}
|
|
}
|
|
|
|
return true;
|
|
}
|
|
|
|
void test_blank_image()
|
|
{
|
|
const gil::point_t dimensions(20, 20);
|
|
gil::gray16s_image_t dx(dimensions, gil::gray16s_pixel_t(0), 0);
|
|
gil::gray16s_image_t dy(dimensions, gil::gray16s_pixel_t(0), 0);
|
|
|
|
gil::gray32f_image_t m11(dimensions);
|
|
gil::gray32f_image_t m12_21(dimensions);
|
|
gil::gray32f_image_t m22(dimensions);
|
|
gil::gray32f_image_t expected(dimensions, gil::gray32f_pixel_t(0), 0);
|
|
gil::compute_tensor_entries(
|
|
gil::view(dx),
|
|
gil::view(dy),
|
|
gil::view(m11),
|
|
gil::view(m12_21),
|
|
gil::view(m22)
|
|
);
|
|
BOOST_TEST(are_equal(gil::view(expected), gil::view(m11)));
|
|
BOOST_TEST(are_equal(gil::view(expected), gil::view(m12_21)));
|
|
BOOST_TEST(are_equal(gil::view(expected), gil::view(m22)));
|
|
|
|
gil::gray32f_image_t harris_response(dimensions, gil::gray32f_pixel_t(0), 0);
|
|
auto unnormalized_mean = gil::generate_unnormalized_mean(5);
|
|
gil::compute_harris_responses(
|
|
gil::view(m11),
|
|
gil::view(m12_21),
|
|
gil::view(m22),
|
|
unnormalized_mean,
|
|
0.04f,
|
|
gil::view(harris_response)
|
|
);
|
|
BOOST_TEST(are_equal(gil::view(expected), gil::view(harris_response)));
|
|
}
|
|
|
|
int main(int argc, char* argv[])
|
|
{
|
|
test_blank_image();
|
|
return boost::report_errors();
|
|
}
|