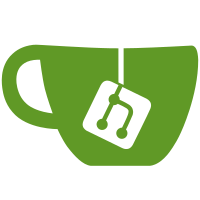
* examples: Sort the examples in the Jamfile. This makes it easier to see which example files are even mentioned in the build. * examples: Build all examples. To at least test that they build with the current API. The newly-mentioned files here seem to be mostly (maybe all) the BGL book examples that were added in 2001:5215e9b4f2
I would prefer to put these all in an examples/bgl_book subdirectory. * Examples build: Comment out examples that cannot be expected to build. For instance, because they depend on SBG or Stanford Graph. * Examples: Add missing <iostream> includes. * Examples: Comment out unused typedefs and variables. * Examples: king_ordering: Adapt to newer API. Specify the extra parameter for king_ordering(). The API was changed in 2005:4bc19a1621
The test code was already correct: https://github.com/imvu/boost/blob/master/libs/graph/test/king_ordering.cpp * Examples: Some graphviz examples: Link to the library. read_graphviz() is not header only. * csr-example: Pass edge_are_sorted to constructor. To use the new (well, in 2009) API:809904f268
* iteration_macros: Use BGL_FORALL_ADJ. Not BGL_FORALL_ADJACENT(), which doesn't exist, because this was changed in 2001:a0061ba07e
* examples: kevin-bacon2: Fix the build. Include boost/serialization/string.hpp to fix this error: kevin-bacon2.cpp:68:9: required from here ../../../boost/serialization/access.hpp:116:9: error: ‘class std::__cxx11::basic_string<char>’ has no member named ‘serialize’ and link to the boost serialization library. * examples: loop_dfs: Add a missing typename keyword. * examples: accum-compile-times: Remove unused variables. * Examples: Remove unused typedefs. * examples: avoid warning about parentheses aronud &&. * example: read_graphviz: Actually use status. * Example: adj_list_ra_edgelist: Fix the build. The [] syntax must have worked once but doesn't anymore. This fixes the build but it is even more clearl now a stupid way to use the edge iterator. * Examples: Remove unused typedefs. * Examples: Remove an unused variable. * Example: iohb: A const correction. Otherwise newer compilers complain about converting string literals to char* when callig this function. * Exmaples: iohb: Avoid security warning with fprintf(). * Examples: Actually use a variable. * Examples: Comment out all Graphviz examples. These use the now-non-existant GraphizGraph and GraphvizDigraph types. Presumably these could be updated but it's not obvious how to do that: https://svn.boost.org/trac/boost/ticket/4762
40 lines
1.2 KiB
C++
40 lines
1.2 KiB
C++
//=======================================================================
|
|
// Copyright 2001 Jeremy G. Siek, Andrew Lumsdaine, Lie-Quan Lee,
|
|
//
|
|
// Distributed under the Boost Software License, Version 1.0. (See
|
|
// accompanying file LICENSE_1_0.txt or copy at
|
|
// http://www.boost.org/LICENSE_1_0.txt)
|
|
//=======================================================================
|
|
#include <boost/config.hpp>
|
|
#include <iostream>
|
|
#include <vector>
|
|
#include <boost/graph/connected_components.hpp>
|
|
#include <boost/graph/adjacency_list.hpp>
|
|
|
|
int
|
|
main()
|
|
{
|
|
using namespace boost;
|
|
typedef adjacency_list < vecS, vecS, undirectedS > Graph;
|
|
|
|
const int N = 6;
|
|
Graph G(N);
|
|
add_edge(0, 1, G);
|
|
add_edge(1, 4, G);
|
|
add_edge(4, 0, G);
|
|
add_edge(2, 5, G);
|
|
|
|
std::vector<int> c(num_vertices(G));
|
|
int num = connected_components
|
|
(G, make_iterator_property_map(c.begin(), get(vertex_index, G), c[0]));
|
|
|
|
std::cout << std::endl;
|
|
std::vector < int >::iterator i;
|
|
std::cout << "Total number of components: " << num << std::endl;
|
|
for (i = c.begin(); i != c.end(); ++i)
|
|
std::cout << "Vertex " << i - c.begin()
|
|
<< " is in component " << *i << std::endl;
|
|
std::cout << std::endl;
|
|
return EXIT_SUCCESS;
|
|
}
|