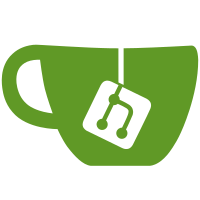
This change excludes boost:: and boost::detail:: namespaces from ADL for unqualified function calls (e.g. algorithms). This reduces the possibility of name clashes with other libraries and user's code. One of the effects should be fixing test failures on gcc 4.2 and 4.4 due to clashed with Boost.TypeTraits. Also some of the functions marked with inline keyword.
86 lines
2.0 KiB
C++
86 lines
2.0 KiB
C++
// (C) Copyright Jens Maurer 2001.
|
|
// Distributed under the Boost Software License, Version 1.0. (See
|
|
// accompanying file LICENSE_1_0.txt or copy at
|
|
// http://www.boost.org/LICENSE_1_0.txt)
|
|
//
|
|
// Revision History:
|
|
|
|
// 15 Nov 2001 Jens Maurer
|
|
// created.
|
|
|
|
// See http://www.boost.org/libs/utility/iterator_adaptors.htm for documentation.
|
|
|
|
#ifndef BOOST_ITERATOR_ADAPTOR_GENERATOR_ITERATOR_HPP
|
|
#define BOOST_ITERATOR_ADAPTOR_GENERATOR_ITERATOR_HPP
|
|
|
|
#include <boost/iterator/iterator_facade.hpp>
|
|
#include <boost/ref.hpp>
|
|
|
|
namespace boost {
|
|
namespace iterators {
|
|
|
|
template<class Generator>
|
|
class generator_iterator
|
|
: public iterator_facade<
|
|
generator_iterator<Generator>
|
|
, typename Generator::result_type
|
|
, single_pass_traversal_tag
|
|
, typename Generator::result_type const&
|
|
>
|
|
{
|
|
typedef iterator_facade<
|
|
generator_iterator<Generator>
|
|
, typename Generator::result_type
|
|
, single_pass_traversal_tag
|
|
, typename Generator::result_type const&
|
|
> super_t;
|
|
|
|
public:
|
|
generator_iterator() {}
|
|
generator_iterator(Generator* g) : m_g(g), m_value((*m_g)()) {}
|
|
|
|
void increment()
|
|
{
|
|
m_value = (*m_g)();
|
|
}
|
|
|
|
const typename Generator::result_type&
|
|
dereference() const
|
|
{
|
|
return m_value;
|
|
}
|
|
|
|
bool equal(generator_iterator const& y) const
|
|
{
|
|
return this->m_g == y.m_g && this->m_value == y.m_value;
|
|
}
|
|
|
|
private:
|
|
Generator* m_g;
|
|
typename Generator::result_type m_value;
|
|
};
|
|
|
|
template<class Generator>
|
|
struct generator_iterator_generator
|
|
{
|
|
typedef generator_iterator<Generator> type;
|
|
};
|
|
|
|
template <class Generator>
|
|
inline generator_iterator<Generator>
|
|
make_generator_iterator(Generator & gen)
|
|
{
|
|
typedef generator_iterator<Generator> result_t;
|
|
return result_t(&gen);
|
|
}
|
|
|
|
} // namespace iterators
|
|
|
|
using iterators::generator_iterator;
|
|
using iterators::generator_iterator_generator;
|
|
using iterators::make_generator_iterator;
|
|
|
|
} // namespace boost
|
|
|
|
#endif // BOOST_ITERATOR_ADAPTOR_GENERATOR_ITERATOR_HPP
|