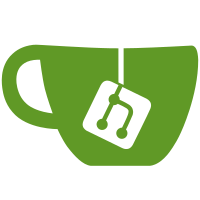
* Added multiple inputs to example In the section after the introduction of using value<vector<...>> to store multiple values, I updated the example to show multiple input values and their results. * Make it clear how to enable sections for ini files It was unclear how the user should support sections, should they have nested options_description's (no), nested variables_map's (no), it is just a dotted string that is input! This adds a snippet showing that. * Added an example for environment options This example shows how to use program_options to pull environmental options into a program. This instance uses a function to map env options to config options. * Added an example showing different types in a config file I went through a lot of the common types that a user may want to include in a config file (especially the boolean options) and showed an example with them all. With some minor modifications, this could also be added to the tests directory as there are several cases in here that I didn't see checked anywhere else in the code. * Added explanation comments to new examples * Added an example with a heirarchy of inputs This file shows an example program that can get inputs from the command line, environmental variables, multiple config files specified on the command line, and a default config file. There are multiple usage examples at the bottom in the comments. * Reference to example showing environment options * Added section detailing type conversion. Added explicity acknowledging that hex/oct/bin formatted strings aren't allowed. Detailed the bool_switch value and what strings evaluate true/false. * Added a global to the config file example * Semicolon typo * Split components into seperate functions * Added unregistered entry and flag to prevent error * Added logic to capture unregistered value * Build new examples * Backslashes need escaping on unix * match permissions
48 lines
1.3 KiB
C++
48 lines
1.3 KiB
C++
// Copyright Thomas Kent 2016
|
|
// Distributed under the Boost Software License, Version 1.0.
|
|
// (See accompanying file LICENSE_1_0.txt
|
|
// or copy at http://www.boost.org/LICENSE_1_0.txt)
|
|
|
|
#include <boost/program_options.hpp>
|
|
namespace po = boost::program_options;
|
|
#include <string>
|
|
#include <iostream>
|
|
|
|
std::string mapper(std::string env_var)
|
|
{
|
|
// ensure the env_var is all caps
|
|
std::transform(env_var.begin(), env_var.end(), env_var.begin(), ::toupper);
|
|
|
|
if (env_var == "PATH") return "path";
|
|
if (env_var == "EXAMPLE_VERBOSE") return "verbosity";
|
|
return "";
|
|
}
|
|
|
|
void get_env_options()
|
|
{
|
|
po::options_description config("Configuration");
|
|
config.add_options()
|
|
("path", "the execution path")
|
|
("verbosity", po::value<std::string>()->default_value("INFO"), "set verbosity: DEBUG, INFO, WARN, ERROR, FATAL")
|
|
;
|
|
|
|
po::variables_map vm;
|
|
store(po::parse_environment(config, boost::function1<std::string, std::string>(mapper)), vm);
|
|
notify(vm);
|
|
|
|
if (vm.count("path"))
|
|
{
|
|
std::cout << "First 75 chars of the system path: \n";
|
|
std::cout << vm["path"].as<std::string>().substr(0, 75) << std::endl;
|
|
}
|
|
|
|
std::cout << "Verbosity: " << vm["verbosity"].as<std::string>() << std::endl;
|
|
}
|
|
|
|
int main(int ac, char* av[])
|
|
{
|
|
get_env_options();
|
|
|
|
return 0;
|
|
}
|