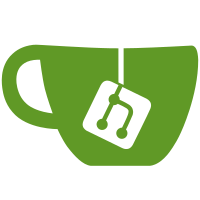
commit a384431cd1ce230630d6a4522022de919cb55ac9 Author: dragonCASTjosh <dragonCASTjosh@gmail.com> Date: Thu Nov 10 00:16:26 2016 +0000 Fixed typing and style errors commit d4f8ca2eeed2b0196b46bd391e96a511594dc738 Author: dragonCASTjosh <dragonCASTjosh@gmail.com> Date: Thu Nov 10 00:14:02 2016 +0000 Removed isAreaLight from attibutes commit 62d50f3ba94d9c665358dcd7377730d968fde7be Author: dragonCASTjosh <dragonCASTjosh@gmail.com> Date: Mon Nov 7 22:53:45 2016 +0000 Added documentation on area lighting options commit 53e5844bee5caccc2a37f0dcdac3b8d4a984dd62 Author: dragonCASTjosh <dragonCASTjosh@gmail.com> Date: Sat Nov 5 17:15:14 2016 +0000 Fixd spacing commit 5afa4ec59c1ecd2aa4892d4854e3b954af9cc73f Author: dragonCASTjosh <dragonCASTjosh@gmail.com> Date: Sat Nov 5 06:00:19 2016 +0000 Fixed materials under deferred and light position in the scene commit 314bc5847a44979b89f46a0272fcf5cd0f04e866 Author: dragonCASTjosh <dragonCASTjosh@gmail.com> Date: Sat Nov 5 05:14:27 2016 +0000 Fixed Deferred rendering commit bde3560de71ab5a33c9cc3a4ee40029567cca5bf Author: dragonCASTjosh <dragonCASTjosh@gmail.com> Date: Sat Nov 5 03:26:54 2016 +0000 Area lighting, improved IBL and improved attenuation for OpenGL commit 52be0fc67626a9c8901091445be52bdbe99657d1 Author: dragonCASTjosh <dragonCASTjosh@gmail.com> Date: Sat Nov 5 01:23:53 2016 +0000 Intergrated Area ligthing with the editor, and improved texture quality commit 5044bebe3d8799dee687ca676334f4493586d376 Author: dragonCASTjosh <dragonCASTjosh@gmail.com> Date: Mon Oct 24 09:25:15 2016 +0100 Tube lighting HLSL commit f3849a93d1812345b21918a7418d12c8d3e1e3dd Author: dragonCASTjosh <dragonCASTjosh@gmail.com> Date: Mon Oct 24 09:01:10 2016 +0100 Improve IBL commit 210bfaebb40ed977eb46f0f6f91b3b6a49ec25a7 Author: joshua Nuttall <dragoncastjosh@live.com> Date: Thu Sep 15 12:32:15 2016 +0100 Made sphere lights closer to epics paper commit c186870e9e79de9572b5302019598ac059af58cf Author: joshua Nuttall <dragoncastjosh@live.com> Date: Thu Sep 15 11:57:39 2016 +0100 Added sphere light and beginning of tube lights
129 lines
4.0 KiB
HLSL
129 lines
4.0 KiB
HLSL
#include "Uniforms.hlsl"
|
|
#include "Samplers.hlsl"
|
|
#include "Transform.hlsl"
|
|
#include "ScreenPos.hlsl"
|
|
#include "Lighting.hlsl"
|
|
#include "Constants.hlsl"
|
|
#include "PBR.hlsl"
|
|
#line 9
|
|
|
|
void VS(float4 iPos : POSITION,
|
|
#ifdef DIRLIGHT
|
|
out float2 oScreenPos : TEXCOORD0,
|
|
#else
|
|
out float4 oScreenPos : TEXCOORD0,
|
|
#endif
|
|
out float3 oFarRay : TEXCOORD1,
|
|
#ifdef ORTHO
|
|
out float3 oNearRay : TEXCOORD2,
|
|
#endif
|
|
out float4 oPos : OUTPOSITION)
|
|
{
|
|
float4x3 modelMatrix = iModelMatrix;
|
|
float3 worldPos = GetWorldPos(modelMatrix);
|
|
oPos = GetClipPos(worldPos);
|
|
#ifdef DIRLIGHT
|
|
oScreenPos = GetScreenPosPreDiv(oPos);
|
|
oFarRay = GetFarRay(oPos);
|
|
#ifdef ORTHO
|
|
oNearRay = GetNearRay(oPos);
|
|
#endif
|
|
#else
|
|
oScreenPos = GetScreenPos(oPos);
|
|
oFarRay = GetFarRay(oPos) * oPos.w;
|
|
#ifdef ORTHO
|
|
oNearRay = GetNearRay(oPos) * oPos.w;
|
|
#endif
|
|
#endif
|
|
}
|
|
|
|
void PS(
|
|
#ifdef DIRLIGHT
|
|
float2 iScreenPos : TEXCOORD0,
|
|
#else
|
|
float4 iScreenPos : TEXCOORD0,
|
|
#endif
|
|
float3 iFarRay : TEXCOORD1,
|
|
#ifdef ORTHO
|
|
float3 iNearRay : TEXCOORD2,
|
|
#endif
|
|
|
|
float2 iFragPos : VPOS,
|
|
out float4 oColor : OUTCOLOR0)
|
|
{
|
|
// If rendering a directional light quad, optimize out the w divide
|
|
#ifdef DIRLIGHT
|
|
float3 depth = Sample2DLod0(DepthBuffer, iScreenPos).r;
|
|
#ifdef HWDEPTH
|
|
depth = ReconstructDepth(depth);
|
|
#endif
|
|
#ifdef ORTHO
|
|
float3 worldPos = lerp(iNearRay, iFarRay, depth);
|
|
#else
|
|
float3 worldPos = iFarRay * depth;
|
|
#endif
|
|
const float4 albedoInput = Sample2DLod0(AlbedoBuffer, iScreenPos);
|
|
const float4 normalInput = Sample2DLod0(NormalBuffer, iScreenPos);
|
|
const float4 specularInput = Sample2DLod0(SpecMap, iScreenPos);
|
|
#else
|
|
float depth = Sample2DProj(DepthBuffer, iScreenPos).r;
|
|
#ifdef HWDEPTH
|
|
depth = ReconstructDepth(depth);
|
|
#endif
|
|
#ifdef ORTHO
|
|
float3 worldPos = lerp(iNearRay, iFarRay, depth) / iScreenPos.w;
|
|
#else
|
|
float3 worldPos = iFarRay * depth / iScreenPos.w;
|
|
#endif
|
|
const float4 albedoInput = Sample2DProj(AlbedoBuffer, iScreenPos);
|
|
const float4 normalInput = Sample2DProj(NormalBuffer, iScreenPos);
|
|
const float4 specularInput = Sample2DProj(SpecMap, iScreenPos);
|
|
#endif
|
|
|
|
// Position acquired via near/far ray is relative to camera. Bring position to world space
|
|
float3 eyeVec = -worldPos;
|
|
worldPos += cCameraPosPS;
|
|
|
|
float3 normal = normalInput.rgb;
|
|
const float roughness = length(normal);
|
|
normal = normalize(normal);
|
|
|
|
const float3 specColor = specularInput.rgb;
|
|
|
|
const float4 projWorldPos = float4(worldPos, 1.0);
|
|
|
|
float3 lightDir;
|
|
float atten = 1;
|
|
|
|
#if defined(DIRLIGHT)
|
|
atten = GetAtten(normal, worldPos, lightDir);
|
|
#elif defined(SPOTLIGHT)
|
|
atten = GetAttenSpot(normal, worldPos, lightDir);
|
|
#else
|
|
atten = GetAttenPoint(normal, worldPos, lightDir);
|
|
#endif
|
|
|
|
float shadow = 1;
|
|
#ifdef SHADOW
|
|
shadow *= GetShadowDeferred(projWorldPos, normal, depth);
|
|
#endif
|
|
|
|
#if defined(SPOTLIGHT)
|
|
const float4 spotPos = mul(projWorldPos, cLightMatricesPS[0]);
|
|
const float3 lightColor = spotPos.w > 0.0 ? Sample2DProj(LightSpotMap, spotPos).rgb * cLightColor.rgb : 0.0;
|
|
#elif defined(CUBEMASK)
|
|
const float3 lightColor = texCUBE(sLightCubeMap, mul(worldPos - cLightPosPS.xyz, (float3x3)cLightMatricesPS[0])).rgb * cLightColor.rgb;
|
|
#else
|
|
const float3 lightColor = cLightColor.rgb;
|
|
#endif
|
|
|
|
const float3 toCamera = normalize(eyeVec);
|
|
const float3 lightVec = normalize(lightDir);
|
|
const float ndl = clamp(abs(dot(normal, lightVec)), M_EPSILON, 1.0);
|
|
|
|
float3 BRDF = GetBRDF(worldPos, lightDir, lightVec, toCamera, normal, roughness, albedoInput.rgb, specColor);
|
|
|
|
oColor.a = 1;
|
|
oColor.rgb = BRDF * lightColor * shadow * atten / M_PI;
|
|
}
|